vue-cli とは
Vue.js プロジェクトのひな形を作るためのコマンドラインツール。 Node.js 上で動作します。
Simple CLI for scaffolding Vue.js projects
— vuejs/vue-cli
インストール
$ npm install -g vue-cli
使い方
$ vue init <template-name> <project-name>
hot reload, linting, testing & css extraction.
$ vue init webpack my-project
for quick prototyping
$ vue init webpack-simple my-project
単一ファイルコンポーネント
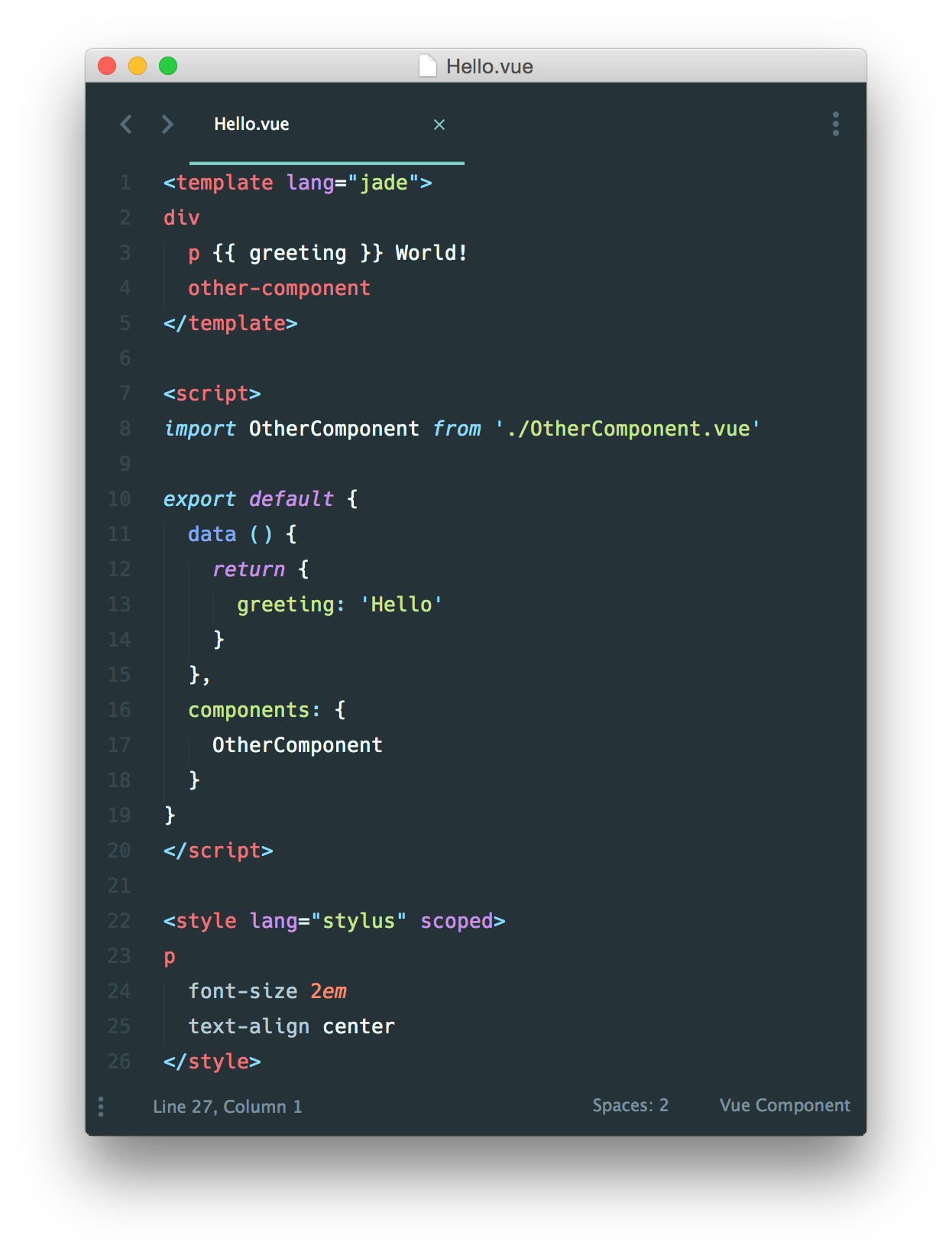
テンプレートを Pug (Jade) で書く
pug
と pug-loader
をインストールします。
$ npm install --save-dev pug pug-loader
<template lang="pug">
div
p {{ greeting }} World!
other-component
</template>
スタイルを Stylus で書く
stylus
と stylus-loader
をインストールします。
$ npm install --save-dev stylus stylus-loader
<style lang="stylus" scoped>
p
font-size 2em
text-align center
</style>
jQueryを使う
jquery
をインストールして webpack にプラグインとして登録します。
$ npm install --save jquery
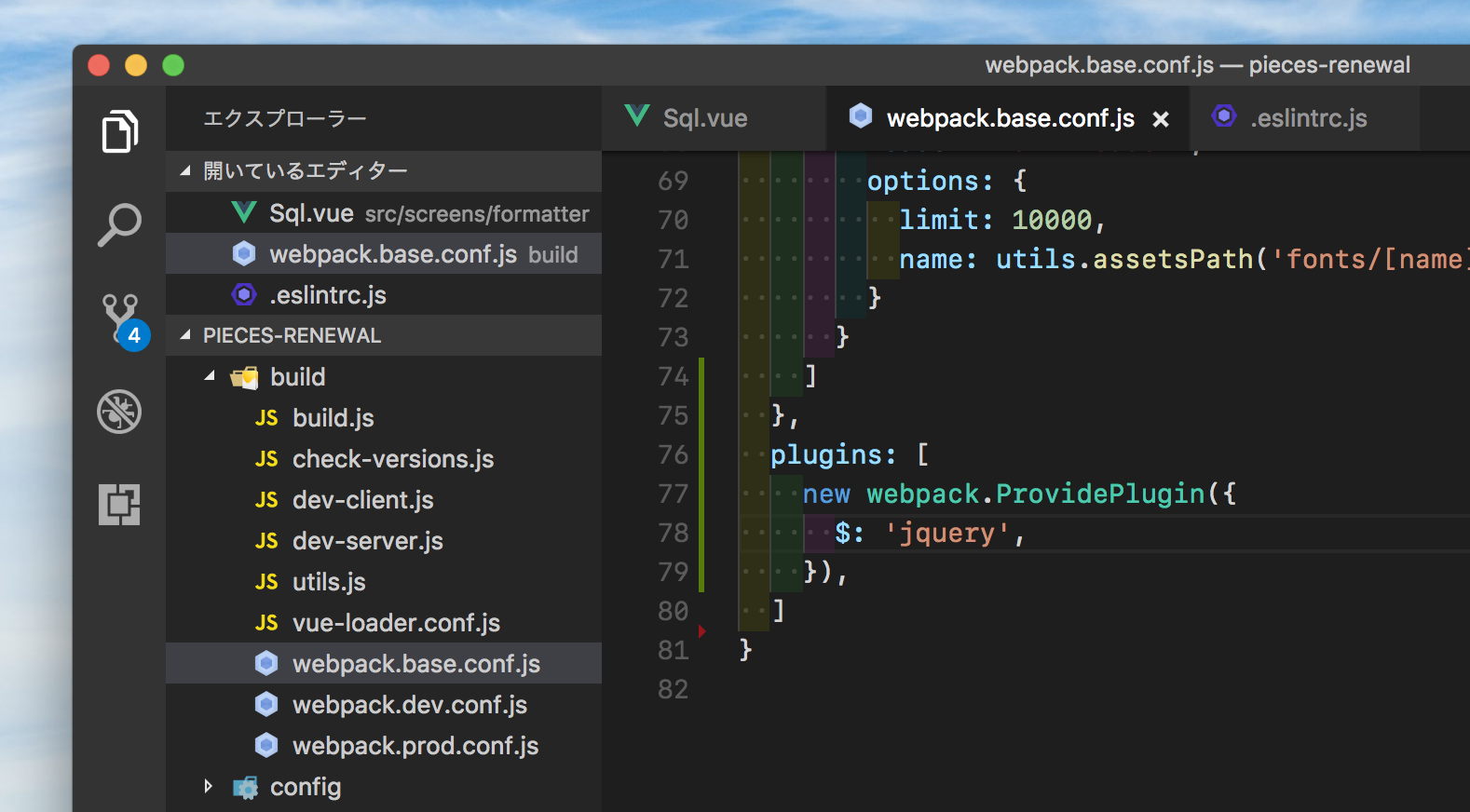
webpack.base.conf.js
const webpack = require('webpack')
// :
plugins: [
new webpack.ProvidePlugin({
$: 'jquery',
}),
]
// :
'$' is not defined`
webpack プラグインだけ登録しても、 ESLint の設定をしないと動作しません。
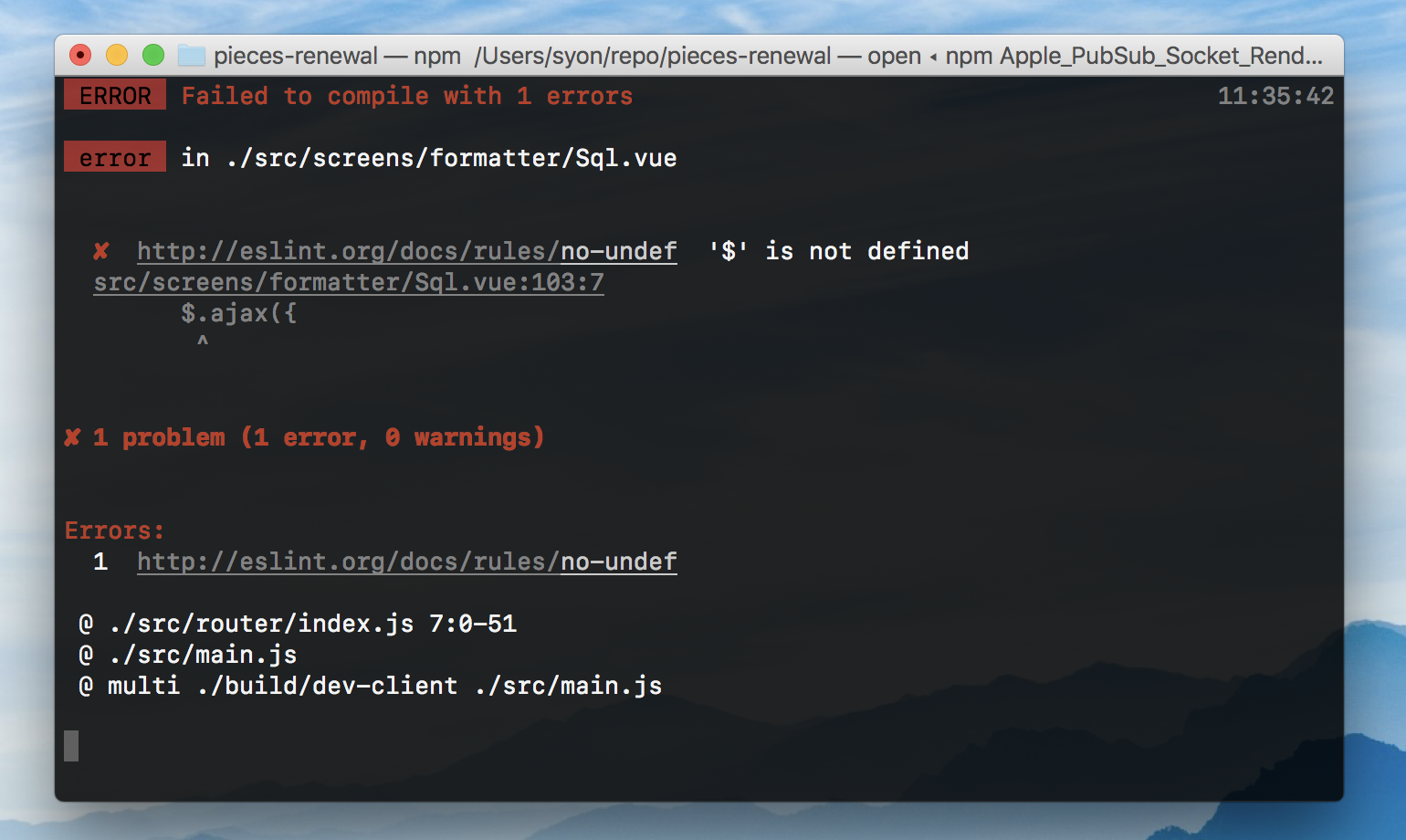
✘ http://eslint.org/docs/rules/no-undef '$' is not defined
ESLint の設定も変更してエラーが出ないようにします。
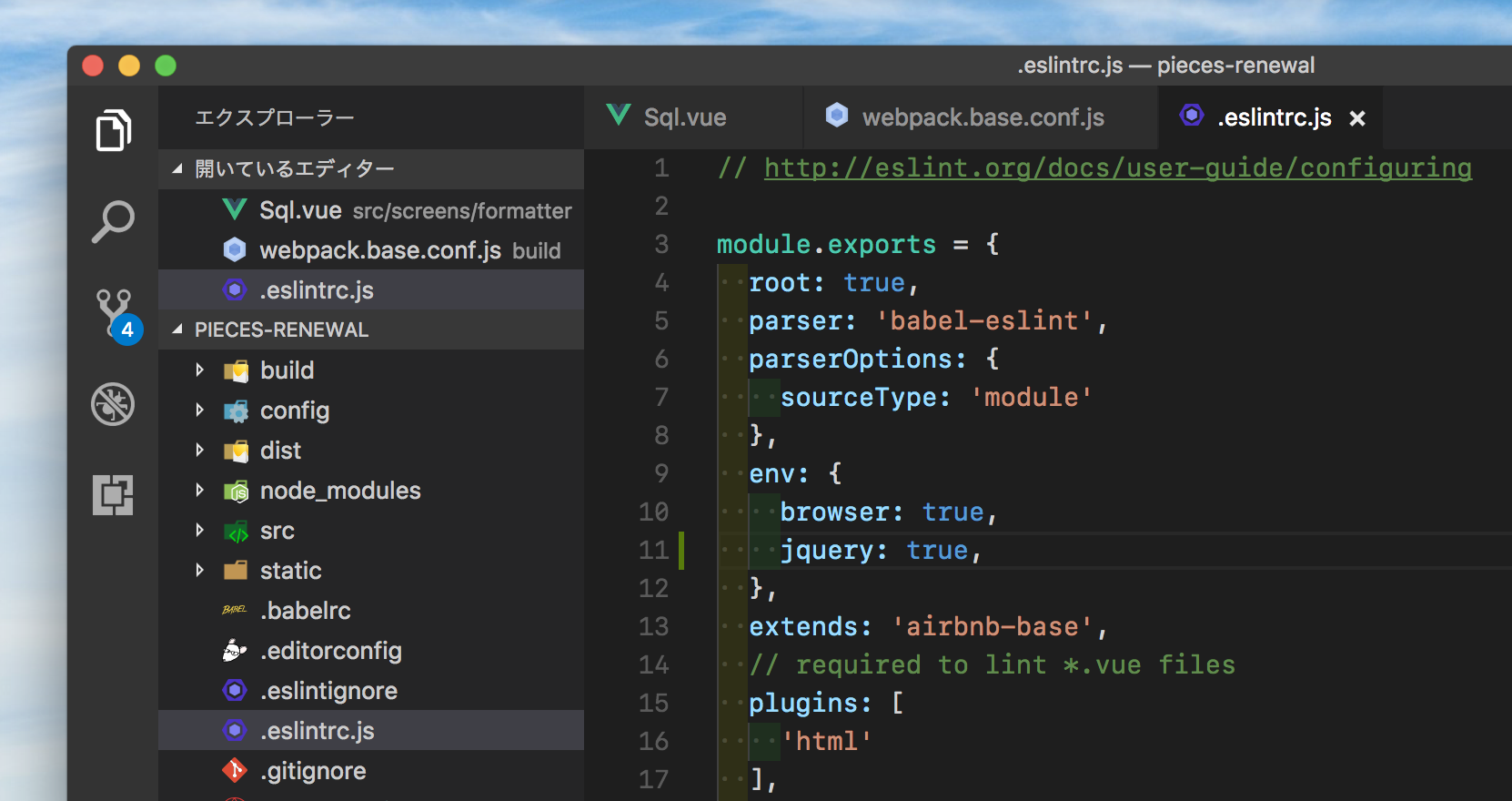
eslintrc.js
env: {
browser: true,
jquery: true,
},
debugを導入する
debug
をインストールして webpack にプラグインとして登録します。
$ npm install --save debug
webpack.base.conf.js
const webpack = require('webpack')
// :
plugins: [
new webpack.ProvidePlugin({
Debug: 'debug',
}),
]
// :
Vuexを導入する
vuex
をインストールします。
$ npm install --save vuex
ストアを扱うコードを作成し、Vueと接続します。
main.js
// :
import store from './store'
// :
new Vue({
el: '#app',
store,
// :
})
store.js
import Vue from 'vue';
import Vuex from 'vuex';
Vue.use(Vuex);
export default new Vuex.Store({
state: {
count: 777,
apiResult: {},
},
mutations: {
increment(state) {
state.count = state.count + 1;
},
setApiResult(state, payload) {
state.apiResult = payload;
},
},
actions: {
increment(context) {
context.commit('increment');
},
setApiResult(context, payload) {
context.commit('setApiResult', payload);
},
},
})
規模が大きくなってきたら、公式のサンプルのようにストアを分割します。
state
コンポーネント内でストアのステートを使うには、1つの例としてこのように記述します。
SomeComponent.vue
<template lang="pug">
h1 {{ count }}
</template>
<script>
export default {
computed: {
count() {
return this.$store.state.count;
},
},
}
</script>
ストアのものをそのままコンポーネントで利用する場合には、上記の書き方では冗長なので 以下のように書くこともできます。
<template lang="pug">
h1 {{ count }}
</template>
<script>
import { mapState } from 'vuex';
export default {
computed: {
...mapState([
'count',
]),
},
}
</script>
actions
SomeComponent.vue
<template lang="pug">
button(@click="doIncrement") Increment
</template>
<script>
import { mapActions } from 'vuex';
export default {
methods: {
...mapActions({
doIncrement: 'increment',
}),
fetchApi() {
// :
this.$store.dispatch('setApiResult', json.list);
},
},
}
</script>
mutations
Vue のリアクティブなルールに則ったミューテーション
新しいプロパティをオブジェクトに追加するとき、以下のいずれかが必要です:
Vue.set(obj, 'newProp', 123)
state.obj = { ...state.obj, newProp: 123 }
エントリーIDをキーに持つ連想配列をマージするようなケースでは、スプレッドシンタックスを使って以下のようにします。
state.entries = { ...state.entries, ...newEntries }
Cookieを扱う
$ npm install --save vue-cookie
main.js
import Vue from 'vue';
import VueCookie from 'vue-cookie';
// :
Vue.use(VueCookie);
// :
computed: {
token: function () {
return this.$cookie.get('token');
},
},
methods: {
getToken() {
// expires in one day
this.$cookie.set('token', 'xxxxxxxxxx', { expires: '1D' });
},
},
IE11 に対応する
Promise
Unhandled promise rejection ReferenceError 'Promise' は定義されていません。
— IE11
$ npm install --save babel-polyfill
main.js
// :
import 'babel-polyfill'
// :
fetch
Unhandled promise rejection ReferenceError 'fetch' は定義されていません。
— IE11
$ npm install --save whatwg-fetch
main.js
// :
import 'whatwg-fetch'
// :
別のホストからIPアドレスで接続して動作確認
bash
$ export HOST=0.0.0.0
$ npm run dev
fish shell
$ set -x HOST 0.0.0.0
$ npm run dev
開発サーバの設定ファイルがいろいろと参考になります。
config/index.js
// Various Dev Server settings
host: 'localhost', // can be overwritten by process.env.HOST
port: 8080, // can be overwritten by process.env.HOST, if port is in use, a free one will be determined
autoOpenBrowser: false,
errorOverlay: true,
notifyOnErrors: true,
poll: false, // https://webpack.js.org/configuration/dev-server/#devserver-watchoptions-
Note
ToDo
-
PostCSS, Autoprefixer
-
eslint-plugin-pug